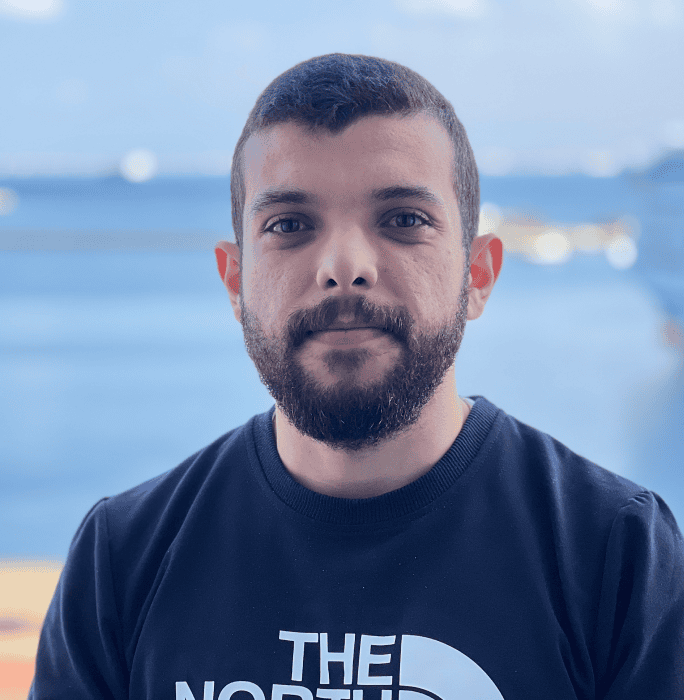
👋 Hey, I'm Ouassim
I'm a software engineer focused on delivering high-quality products. I enjoy working where design and engineering intersect, building software that's visually appealing and technically solid.
This is my personal space, where I share my work, projects and some notes I write here and there.
Work
I focus on getting things done quickly without compromising quality. I believe in building long-lasting foundations, balancing fast delivery with the long-term value of organized, well-documented code.
Software Engineer
Jan. 2025 - current
Full stack Engineer
Nov. 2024 - Dec. 2024
Lead Software Engineer
Sep. 2022 - Oct. 2024
Projects
hookcn
Collection of re-usable react hooks inspired by shadcn/ui.
shadcn form builder
WYSIWYG shadcn form builder.
ouassim.tech
This awesome website that you're on.
Notes
Lens 📷
I have two beautiful German Shepherds, Sam and G (father and son), who are my favorite photography subjects. I love capturing moments of beauty whenever I see them.
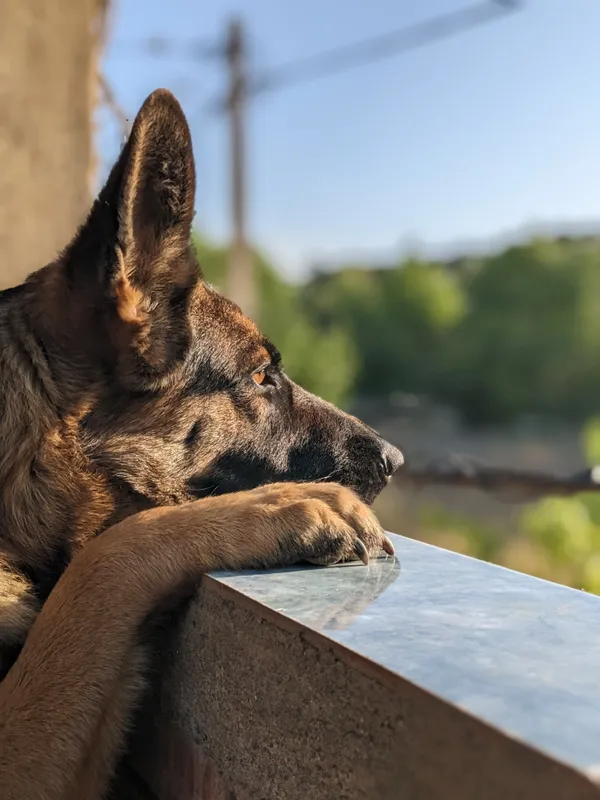
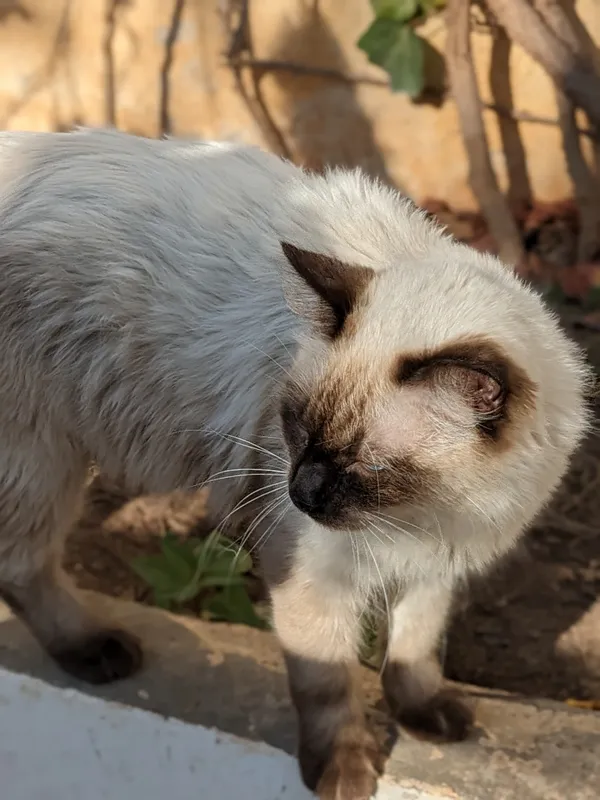
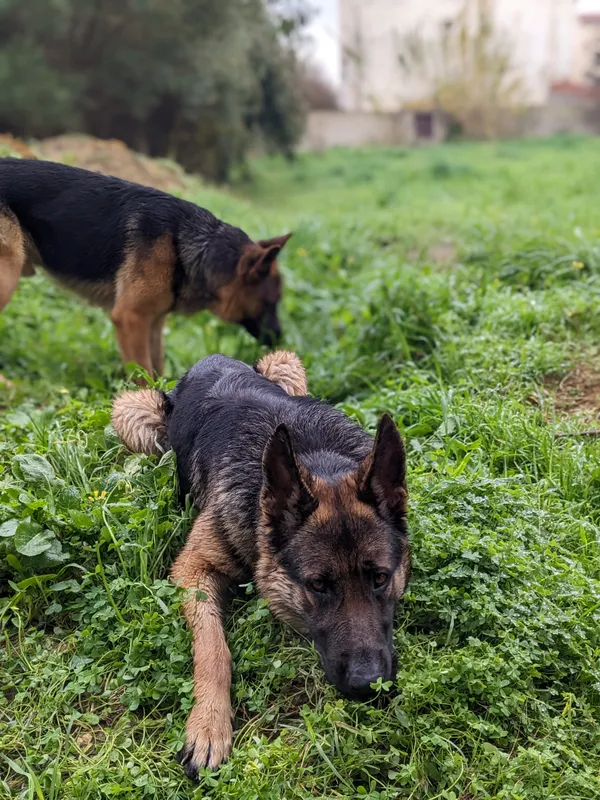
Let's connect
If you want to get in touch with me about something or just to say hi, reach out on social media or send me an email.